HC-SR04超音波センサーを使用して距離を測定するコードです。This code uses the HC-SR04 ultrasonic sensor to measure distance.
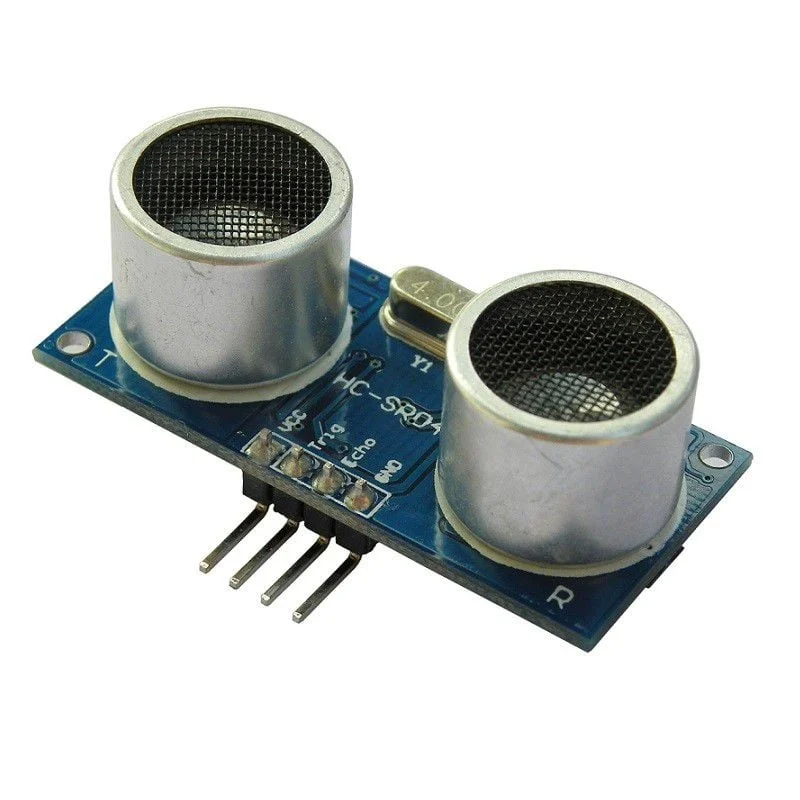
from machine import Timer, Pin
import time
# Define GPIO pin numbers for TRIG and ECHO
TRIG_PIN = 17 # TRIG connected to GPIO17
ECHO_PIN = 16 # ECHO connected to GPIO16
# Initialize TRIG and ECHO pins
TRIG = Pin(TRIG_PIN, Pin.OUT) # Set TRIG pin as output
ECHO = Pin(ECHO_PIN, Pin.IN) # Set ECHO pin as input
def distance():
"""
Measures the distance using the HC-SR04 ultrasonic sensor.
Returns:
float: Distance in millimeters if successful
-1: if measurement fails (timeout)
"""
# Send a 10us pulse to trigger the ultrasonic burst
TRIG.low()
time.sleep_us(2)
TRIG.high()
time.sleep_us(10)
TRIG.low()
# Wait for ECHO to go HIGH (start of echo pulse)
timeout_start = time.ticks_us()
while not ECHO.value():
if time.ticks_diff(time.ticks_us(), timeout_start) > 30000:
return -1 # Timeout: no echo received
# Record the start time of echo pulse
time1 = time.ticks_us()
# Wait for ECHO to go LOW (end of echo pulse)
while ECHO.value():
if time.ticks_diff(time.ticks_us(), time1) > 30000:
return -1 # Timeout: echo pulse too long
# Record the end time of echo pulse
time2 = time.ticks_us()
# Calculate the duration of the echo pulse
duration = time.ticks_diff(time2, time1)
# Calculate distance in millimeters
# Speed of sound = 343 m/s = 343,000 mm/s
# Divide by 2 since the pulse travels to and from the object
distance_mm = duration * 343 / 2 / 1000
return distance_mm
def measure_distance(timer):
"""
Timer callback function that measures and prints the distance.
"""
dis = distance()
if dis != -1:
print("Distance: %.0f mm" % dis)
else:
print("Measurement failed")
# Initialize a timer to call measure_distance() every 2 seconds
timer = Timer()
timer.init(period=2000, mode=Timer.PERIODIC, callback=measure_distance)
import machine
import time
# Define GPIO pins for the ultrasonic sensor
TRIG = machine.Pin(17, machine.Pin.OUT) # TRIG pin (output)
ECHO = machine.Pin(16, machine.Pin.IN) # ECHO pin (input)
def distance():
"""
Measures distance using HC-SR04 with timeout protection.
Returns:
float: Distance in centimeters, or -1 if timeout occurs.
"""
# Send a 10µs pulse to trigger the sensor
TRIG.low()
time.sleep_us(2)
TRIG.high()
time.sleep_us(10)
TRIG.low()
timeout_start = time.ticks_us()
# Wait for ECHO to go HIGH (pulse starts)
while not ECHO.value():
if time.ticks_diff(time.ticks_us(), timeout_start) > 30000:
return -1 # Timeout after 30 ms (no echo start)
time1 = time.ticks_us()
# Wait for ECHO to go LOW (pulse ends)
while ECHO.value():
if time.ticks_diff(time.ticks_us(), time1) > 30000:
return -1 # Timeout after 30 ms (pulse too long)
time2 = time.ticks_us()
# Calculate pulse duration
duration = time.ticks_diff(time2, time1)
# Convert to distance in cm (speed of sound = 340 m/s)
return duration * 340 / 2 / 1000 # distance in mm
# Main loop
while True:
dis = distance()
if dis != -1:
print("Distance: %.0f mm" % dis)
else:
print("Measurement failed (timeout)")
time.sleep_ms(1000)
参考にさせていただいたサイトです。
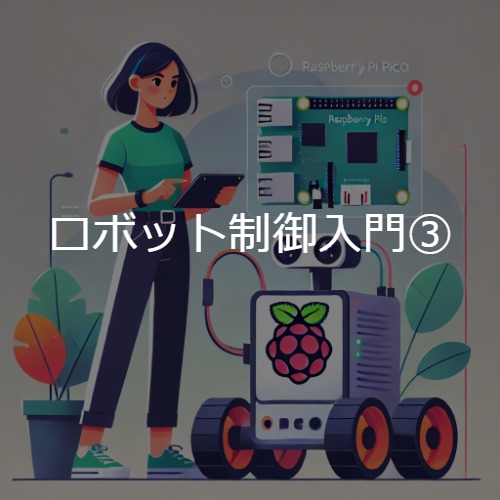
【Raspberry Pi Pico】ロボット制御入門③~I2C通信と障害物回避~【Python】
前回はライブラリの作成について学びました。 今回はセンサー入力を使って自動制御の一旦を体験して
コメント